
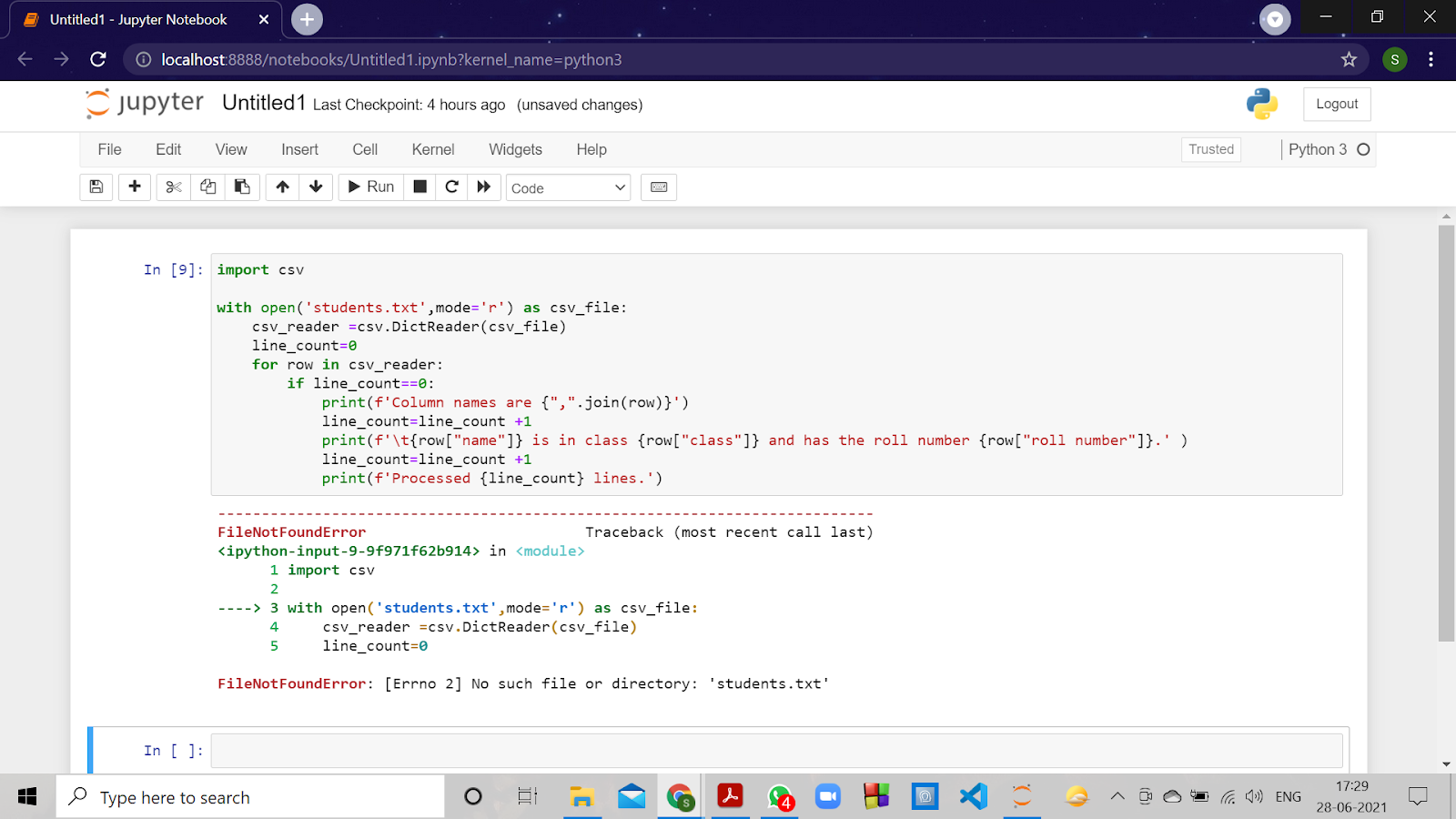
The simplest option would be to use the list function: with open(filename) as f:
#Python read file how to#
Now that you know how to open the file and read it, it's time to store the contents in a list. rstrip() will also take care of the \r! Store the contents as list However if the lines end with \r\n (Windows "newlines") that. One could check if it ends with a trailing newline and if so remove it: with open(filename) as f:īut you could simply remove all whitespaces (including the \n character) from the end of the string, this will also remove all other trailing whitespaces so you have to be careful if these are important: with open(filename) as f:
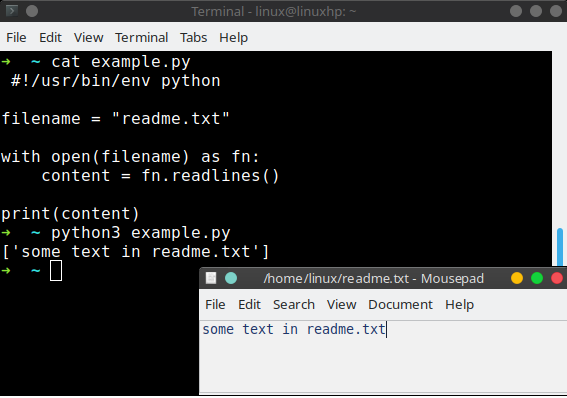
If you don't want that you can could simply remove the last character (or the last two characters on Windows): with open(filename) as f:īut the last line doesn't necessarily has a trailing newline, so one shouldn't use that.
#Python read file mac#
Note however that each line will contain a newline character \n at the end (you might want to check if your Python is built with universal newlines support - otherwise you could also have \r\n on Windows or \r on Mac as newlines). Each iteration will give you a line: with open(filename) as f: The open function returns a file object and it supports Pythons iteration protocol. Okay, you've opened the file, now how to read it?

The last approach is the recommended approach to open a file in Python! Reading the file # The file is always closed after the with-scope ends. However Python provides context managers that have a prettier syntax (but for open it's almost identical to the try and finally above): with open(filename) as f: You could avoid that by using a try and finally: f = open(filename) That will fail to close the file when something between open and close throws an exception. Otherwise it will keep an open file-handle to the file until the process exits (or Python garbages the file-handle). Now that I've shown how to open the file, let's talk about the fact that you always need to close it again. On other platforms the 'b' (binary mode) is simply ignored. In case you want to read in a binary file on Windows you need to use the mode rb: open(filename, 'rb') There is an excellent answer if you want an overview.įor reading a file you can omit the mode or pass it in explicitly: open(filename)īoth will open the file in read-only mode. That's exactly what you need in your case.īut in case you actually want to create a file and/or write to a file you'll need a different argument here. The second argument is the mode, it's r by default which means "read-only". are hidden by default when viewed in the explorer. This is especially important for Windows users because file extensions like. Note that the file extension needs to be specified. Open('/usr/local/afile') # absolute path (linux) Open('C:/users/aname/afile') # absolute path (windows) Open('adir/afile') # relative path (relative to the current working directory) For example: open('afile') # opens the file named afile in the current working directory The filename should be a string that represents the path to the file. Buffering (I'll ignore this argument in this answer).The most commonly used function to open a file in Python is open, it takes one mandatory argument and two optional ones in Python 2.7: I assume that you want to open a specific file and you don't deal directly with a file-handle (or a file-like-handle). To read a file into a list you need to do three things:įortunately Python makes it very easy to do these things so the shortest way to read a file into a list is: lst = list(open(filename)) Print("lines_in_textfile =", lines_in_textfile) Or: > x = open("myfile.txt").read().splitlines() We can use this Python script in the same directory of the txt above > with open("myfile.txt", encoding="utf-8") as file: This is typically how generic parsers will work. This will work nicely for any file size and you go through your file in just 1 pass. (The implementation of the Superman class is left as an exercise for you). Where you define your process function any way you want. So if you process your lines after this, it is not efficient (requires two passes rather than one).Ī better approach for the general case would be the following: with open('/your/path/file') as f: Even if it's not large, it is simply a waste of memory.Ģ) This does not allow processing of each line as you read them. The file could be very large, and you could run out of memory. In the general case, this is a very bad idea. You could simply do the following, as has been suggested: with open('/your/path/file') as f:ġ) You store all the lines in memory.
